The output of lsusb -v will reveal that the device has a subsystem ID of: 0fe9:d501 Making it work Firmware. It requires the firmware file: dvb-usb-bluebird-01.fw Drivers. The following drivers are needed for this device: - lgdt330x.ko - dvb-usb.ko - dvb-usb-cxusb.ko External Links. DViCO product page. All current software, firmware, and drivers for the Surface Pro 3; including optional WinTab drivers. Links to drivers for other Surface devices are in the Details section below. 04 Microsoft® OLE DB Driver 18 for SQL Server® This page is no longer maintained.
In Internet Explorer, click Tools, and then click Internet Options. On the Security tab, click the Trusted Sites icon. Click Sites and then add these website addresses one at a time to the list: You can only add one address at a time and you must click Add after each one. For more info about the Surface MSI naming convention, see Deploy the latest firmware and drivers for Surface devices. If there's not an.msi file that corresponds to the build of Windows 10 you have installed, select the.msi file that's closest to (but still lower than) your build number. Select the “Let me pick from a list of device drivers on my computer” option. Select “Show All Devices” in the drop-down menu. Click on “Have Disk” option. Enter the storage location for the Google USB driver. Click on “Android ADB Interface” from the list.
1.1. The simplest example¶
Here comes a very simple example of an input device driver. The device hasjust one button and the button is accessible at i/o port BUTTON_PORT. Whenpressed or released a BUTTON_IRQ happens. The driver could look like:
1.2. What the example does¶
First it has to include the <linux/input.h> file, which interfaces to theinput subsystem. This provides all the definitions needed.
In the _init function, which is called either upon module load or whenbooting the kernel, it grabs the required resources (it should also checkfor the presence of the device).
Then it allocates a new input device structure with input_allocate_device()and sets up input bitfields. This way the device driver tells the otherparts of the input systems what it is - what events can be generated oraccepted by this input device. Our example device can only generate EV_KEYtype events, and from those only BTN_0 event code. Thus we only set thesetwo bits. We could have used:
as well, but with more than single bits the first approach tends to beshorter.
Then the example driver registers the input device structure by calling:
This adds the button_dev structure to linked lists of the input driver andcalls device handler modules _connect functions to tell them a new inputdevice has appeared. input_register_device() may sleep and therefore mustnot be called from an interrupt or with a spinlock held.
While in use, the only used function of the driver is:
which upon every interrupt from the button checks its state and reports itvia the:
call to the input system. There is no need to check whether the interruptroutine isn’t reporting two same value events (press, press for example) tothe input system, because the input_report_* functions check thatthemselves.
Then there is the:
call to tell those who receive the events that we’ve sent a complete report.This doesn’t seem important in the one button case, but is quite importantfor for example mouse movement, where you don’t want the X and Y valuesto be interpreted separately, because that’d result in a different movement.
1.3. dev->open() and dev->close()¶
In case the driver has to repeatedly poll the device, because it doesn’thave an interrupt coming from it and the polling is too expensive to be doneall the time, or if the device uses a valuable resource (eg. interrupt), itcan use the open and close callback to know when it can stop polling orrelease the interrupt and when it must resume polling or grab the interruptagain. To do that, we would add this to our example driver:
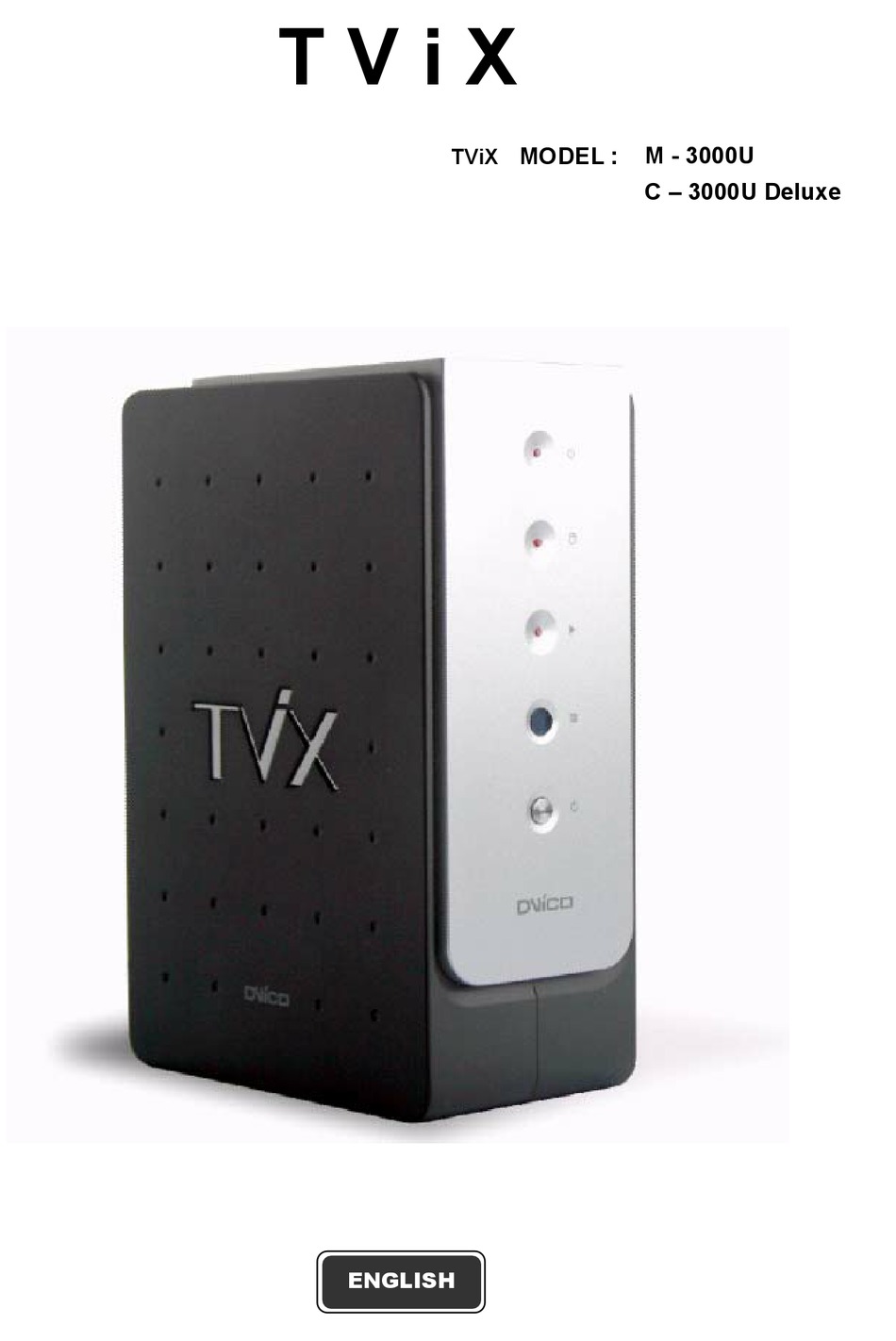
Note that input core keeps track of number of users for the device andmakes sure that dev->open() is called only when the first user connectsto the device and that dev->close() is called when the very last userdisconnects. Calls to both callbacks are serialized.
The open() callback should return a 0 in case of success or any nonzero valuein case of failure. The close() callback (which is void) must always succeed.
1.4. Basic event types¶
The most simple event type is EV_KEY, which is used for keys and buttons.It’s reported to the input system via:
See uapi/linux/input-event-codes.h for the allowable values of code (from 0 toKEY_MAX). Value is interpreted as a truth value, ie any nonzero value means keypressed, zero value means key released. The input code generates events onlyin case the value is different from before.
In addition to EV_KEY, there are two more basic event types: EV_REL andEV_ABS. They are used for relative and absolute values supplied by thedevice. A relative value may be for example a mouse movement in the X axis.The mouse reports it as a relative difference from the last position,because it doesn’t have any absolute coordinate system to work in. Absoluteevents are namely for joysticks and digitizers - devices that do work in anabsolute coordinate systems.
Having the device report EV_REL buttons is as simple as with EV_KEY, simplyset the corresponding bits and call the:
function. Events are generated only for nonzero value.
However EV_ABS requires a little special care. Before callinginput_register_device, you have to fill additional fields in the input_devstruct for each absolute axis your device has. If our button device had alsothe ABS_X axis:
Or, you can just say:
This setting would be appropriate for a joystick X axis, with the minimum of0, maximum of 255 (which the joystick must be able to reach, no problem ifit sometimes reports more, but it must be able to always reach the min andmax values), with noise in the data up to +- 4, and with a center flatposition of size 8.
If you don’t need absfuzz and absflat, you can set them to zero, which meanthat the thing is precise and always returns to exactly the center position(if it has any).
1.5. BITS_TO_LONGS(), BIT_WORD(), BIT_MASK()¶
These three macros from bitops.h help some bitfield computations:
1.6. The id* and name fields¶
The dev->name should be set before registering the input device by the inputdevice driver. It’s a string like ‘Generic button device’ containing auser friendly name of the device.
The id* fields contain the bus ID (PCI, USB, ...), vendor ID and device IDof the device. The bus IDs are defined in input.h. The vendor and device idsare defined in pci_ids.h, usb_ids.h and similar include files. These fieldsshould be set by the input device driver before registering it.
The idtype field can be used for specific information for the input devicedriver.
The id and name fields can be passed to userland via the evdev interface.
1.7. The keycode, keycodemax, keycodesize fields¶
These three fields should be used by input devices that have dense keymaps.The keycode is an array used to map from scancodes to input system keycodes.The keycode max should contain the size of the array and keycodesize thesize of each entry in it (in bytes).
Userspace can query and alter current scancode to keycode mappings usingEVIOCGKEYCODE and EVIOCSKEYCODE ioctls on corresponding evdev interface.When a device has all 3 aforementioned fields filled in, the driver mayrely on kernel’s default implementation of setting and querying keycodemappings.
1.8. dev->getkeycode() and dev->setkeycode()¶
getkeycode() and setkeycode() callbacks allow drivers to override defaultkeycode/keycodesize/keycodemax mapping mechanism provided by input coreand implement sparse keycode maps.
1.9. Key autorepeat¶
... is simple. It is handled by the input.c module. Hardware autorepeat isnot used, because it’s not present in many devices and even where it ispresent, it is broken sometimes (at keyboards: Toshiba notebooks). To enableautorepeat for your device, just set EV_REP in dev->evbit. All will behandled by the input system.
1.10. Other event types, handling output events¶
The other event types up to now are:
- EV_LED - used for the keyboard LEDs.
- EV_SND - used for keyboard beeps.
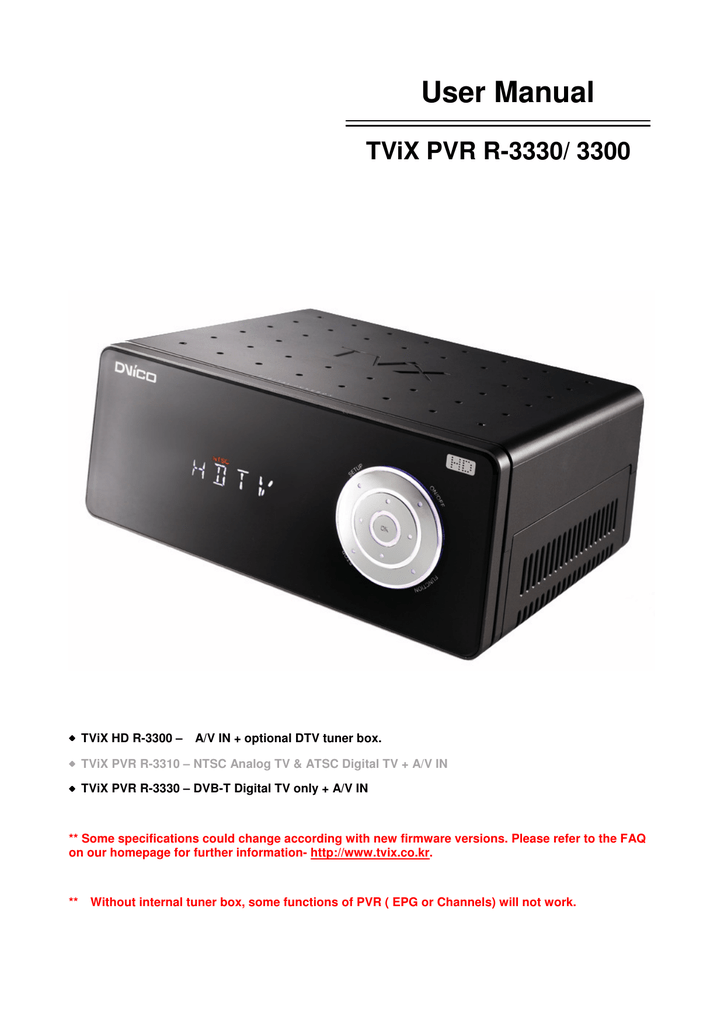
They are very similar to for example key events, but they go in the otherdirection - from the system to the input device driver. If your input devicedriver can handle these events, it has to set the respective bits in evbit,and also the callback routine:
This callback routine can be called from an interrupt or a BH (although thatisn’t a rule), and thus must not sleep, and must not take too long to finish.
Cleanup: This page should be cleared off in preparation for deletion. Tuner specific information should be migrated to the LinuxTV Wiki, and any additional information moved to more generic pages. Discuss the issue on the talk page
- 2Ubuntu 6.10 Installation
- 2.3Hardware
- 2.4Kernel / Packages
- 2.10Remote Control (Lircd)
- 2.12TV Out Setup
- 2.12.2ATI Radeon
The DViCO Dual Digital 2 works out of the box in Ubuntu 10.04, except you'll need to 'enable proprietary drivers' for the DVB firmware.
Note: Ubuntu 9.10 version at: DViCO_FusionHDTV_DVB-T_Dual_Digital_4_Installation
This documentation is my working notes for installing the DViCO FusionHDTV DVB-T Dual Digital onto an Ubuntu 6.10 (Edgy Eft) Distribution. Having had experience and frustrations with the Mythdora and Knoppmyth distros I had to start from scratch on Ubuntu and in my mind has been the easiest way.
Before you Start
Its a good idea sure your graphics card is installed and you can run glxgears:http://ubuntuguide.org/wiki/Ubuntu_Edgy#How_to_install_Beta_Graphics_Driver_.28NVIDIA.29
Card Source
Living on the Gold Coast in Queensland Australia, I picked up the card from http://www.gamedude.com.au. An alternate source is http://www.umart.com.au.
Hardware
Sound
When installing your card, ensure that you plug the dvico audio-out port into the CD-in port (or AUX, if you have one), on your sound card or directly into your motherboard if it has onboard sound. This is an on-card port in both cases - not one of the ones at the rear that you can access from outside your computer. A suitable cable is supplied with the Dual Digital.
2nd USB Tuner and Remote
The 'DViCO FusionHDTV DVB-T Dual Tuner' card has two tuners. One is PCI, the other is USB. You will either need to connect an external USB cable to your card, or use the supplied internal USB cable which connects to your motherboard. This will enable the second tuner and is needed for the remote to function.
Kernel / Packages
After installing Ubuntu Edgy do a full update. Edgy will prompt you to install updates as soon as its loaded. I ended up with the kernel 2.6.17-11-generic after a reboot. As soon as you have rebooted open a terminal and type dmesg to see that the PCI portion at least has been detected.
You should also see the directory /dev/dvb/adapter0
Firmware
The card requires firmware to operate. To get the firmware to the following:
USB Problem
I have had a problem with the usb which may be fixed in the next release of Ubuntu. Let me step you through it if you get this problem.
1. unplug and replug your usb
Looking at the error I noticed ehci_hcd so for the hell of it - I removed it!
In this dmesg you can see that it found our firmware that we downloaded and changed our USB from a cold state to a warm state. However it told us that it needs the usb2.0 drivers to work properly. So we will reload the module that we removed before.
So there you have it, USB second tuner fully loaded and the remote is now working too. You should now have:
To do this automatically on startup add this to your rc.local file
Also notice in the last dmesg that the IR receiver was registered on /class/input/input3. To test the remote do:
Now when you press a button on the remote you should see gibberish appear. This proves the remote is working.
Installing MythTV
- Go to: System > Administration > Synaptic, then select repositories, tick universe & multiverse then close. Click on reload, to reload the sources. Do all updates.
- Open synaptic again (typing synaptic at the terminal or Administration>Synaptic), search for 'mysql-server' (should be mysql-server 5), right click on it and 'mark to install', click apply and yes to all dependencies. Wait for it to finish.
- Stay in Synaptic and search 'mythtv', then mark for installation, mythtvplugins, mythtv, mythtv-themes. Yes to all.
- After installation mythtv asks for a mysql password, just hit enter for both pop-ups. If you screw this up (like i did) go back to synaptic and search again for myuthtv and press Ctrl-A to select all, Right Click and Mark for complete removal. Then reinstall them.
Set up Myth TV
This is my quick and dirty setup. Leave the defaults for everything else (ie Next.. Next.. etc Finish).
- Run mythtv-setup
- Go into General > On the third page:
- Go into Capture Card > New Capture Card
- Go into Video Sources > New Video Source
- Go Into Input connections > DVB:0
You should see all your channels being found. Wait for it to finish and we will proceed to the next step. If you want to come back here and your myth backend is running you can always stop and start the backend by using these commands:
Getting Your Australian Channel Listings
My personal preference is to head over to http://www.oztivo.net/twiki/bin/view/Guidedata/WebHome and Sign Up.After that is done click on the TV Guide web interface and choose your channel listings that you wish to download. For me that was: 7TWO, ABC-Qld, ABC2, GO, Nine-Qld, One-Qld, SBS-Qld, SBSTWO-Qld, Seven-Qld, Ten-Qld
Now we need to make a script to download our listings each day and put it in our database:
Paste the following into tvlistings_tvg and replace username:password with your tvguide username and password.
Save and close and make the script runable, and check to see that it works:
For additional reading about this check out TvGuides HOWTO for MythTV
MythWeb Setup
After you ran tvlisting_tvg the mythfilldatabase section should of complained that it couldn't find certain channels. This is because we haven't mapped the XMLID of the tvlisting channels to the scanned channels. The easiest way to do this is through mythweb.
To get mythweb going you will need to do a few things firstly change the permissions on the directories:
Next you will have to change the password in the mythweb-htaccess.conf file. So open up both files.
Cut and paste the password from mysql.txt into mythweb-htaccess.conf Line 53.
Then open up a browser and go to http://localhost/mythweb/ to check your work.
Map the XMLTVID
You can map this in mythtv-setup but it is far easier to do it in mythweb.
- Open Myththweb > Settings (icon with the key and spanner) > MythTV channel info
- I mapped the following XMLTVID's:
You can change the visibility of the other scanned channels too if you want.In a terminal see if your daily cron job works now:
If that worked then you can go back to mythweb and check out your program listings :)
Remote Control (Lircd)
This I found to be the most painful as I was using the wrong driver for a long time. But after i switched it became a breeze. Now on my system i have 2 /dev/input/events that work when you press a button. When i do a cat /dev/input/event3 and press the 0 key on the remote this happens:
So it seems that this should be the one to use.... Unfortunately Not! Something else is parsing the input from your remote and is producing that. What you really want is something that produces binary gibberish when you press a button:
If your screen is mangled from catting out that then you can type 'reset' to reset your terminal. So now that we have determined what device we should use it is time to see if we can record our key presses. We will be using the driver dev/input and not the driver dvico like i did for way to many days.Another thing that may stop the following from working is if lircd is running. You can stop it by '/etc/init.d/lirc stop'.
You must get dots when holding down a key and nothing else. If you don't get dots or other symbols are showing up then you either have a driver problem or are using the wrong input. Otherwise bob.txt will hold all your keys. Also I found that it wants to set the toggle bit to 1 in bob.txt. This is wrong as you will most likely get multiple keypresses sent. Set toggle_bit=0.
Sometimes irrecord sets an incorrect toggle_bit_mask as well. Mine was set to 0x80000000 and I suffered from duplicate events on every keypress. Changing it to toggle_bit_mask 0x16000000 fixed this problem for me.
If you have come this far then your remote is working like a charm. Time to map the keys and make lircd run automatically. This is done with 3 files:
Make sure you modify your DRIVER and DEVICE to be the same as you used for irrecord. I also changed START_LIRCMD=false for some reason.
/etc/lirc/hardware.conf
Ok since we have set up these two files lets check our work.
lircd is running with the correct driver and device. Ok let run irw and press some keys (Up, Ok and Start) on our remote
Great Now we have to map these keys so that mythtv can use them. which brings us to our final file lircrc. You should save this file in your user directory as it is for personal mappings of the remote.
lircrc
Drivers Dvico Input Devices Definition
Remote Not Working after reboot
Now you may run into this as I did. I rebooted my computer without my USB mouse plugged in and my remotes device /dev/input/event3 had changed to /dev/input/event2. So we now need a way to make sure that the IR is always the same device. A very simple solution is to look at its name in dmesg:
Its name is IR-receiver inside an USB DVB receiver so we take that name and edit the udev rules to create a symlink each time it is plugged in. Add the following to the bottom of the file:
No reboot is nesessary just unplug and replug the USB. You should now have /dev/input/irremote. So now change your hardware.conf to this new device and restart lirc:
Make your power button start MythTV
Taken from the FedoraMyth Tips and Tricks Section.To start and stop your mythfrontend using your remote first you must enable irexec to run in the background. This is simply done using the command:
We will add this to our /etc/rc.local file to have this start on boot:
/etc/rc.local
Now we need to create a mythpowerbutton.sh script in your /usr/local/bin to start and kill the mythfrontend process:
And dont forget to make it executable:
Next edit your ~/.lircrc file to bind your power button. Add the following lines:
~/.lircrc
Now restart lircd to reread your file and restart the irexec daemon (beacuse lircd will shut it down):
Now Press your power button (wait a few moments for it to load) and MythTV frontend should spring into view. Press it again and it sould kill the process. Perfect wife/girlfriend proof restart procedure.
VLC DVD and Media Player
I Like VLC as it has never failed to play something i have in Linux or Windows. I did try xine but it failed to play a Divx 5 film that i had. So I went for gold old VLC. It was surprisingly easy to configure too:
- Synaptic > Search for VLC > Download and Install.
- To Set up your remote paste the following into your local .lircrc file (NOT .mythtv/lircrc):
- Finally run mythfrontend > Utilities/Setup > Setup > Media Settings > Video Settings > Player Settings
- Set VLC to run fullscreen with remote: vlc --control=lirc -f %s
If you want to know what keys you can use for this config file check out: VLC - Lirc Keys. If you just want to use vlc for the show you cant use in xine or mplayer then there is an option to use a separate player for each video.
TV Out Setup
NVIDIA
My TV Out setup was pretty simple I changed my /etc/X11/xorg.conf file so that my screen would be extended to the right.
Important Point: Remember after changing your xconf file that you reboot with your TV ON and PLUGGED IN to your computer!
When your computer boots typically you will see the boot splash on both screens. When the login page appears you may see a NVIDIA logo on the tv and then it will go black. When you log in the TV will light back up.
There are some good tutorials on this so check out: Separate x-screens on Monitor and TVHOWTO TV-Out
The interesting parts of my /etc/X11/xorg.conf:
/etc/X11/xorg.conf
ATI Radeon
Warning: Overall I do not recommend this option at all. It is time consuming and very frustrating to get this to work and even then I was still getting segfaults and unexpected mythbackend crashes. Myth should be as reliable as possible so I recommend that you get yourself a nice cheap NVidia card (The extra $20 will save you days of work). In the end I gave up and will be going back to a Nvidia card. You have been warned!
Note: AMD has released 900+ Pages Of ATI GPU Specs (12th September 2007) - so decent linux support for ATI is finally coming
I recently switched out my card from my expensive NVidia to an cheap ($50) Ati X300 with TV out. It turned out to be a major pain in the ass. All over the place I was getting slow framerates with mythfrontend messages saying my system cannot cope with the current 800x600 output?!. Anyway after about 10hours of mucking around with the thing I finally got a Semi working config. Dual screens and running 1280x1024 on my LCD and 800x600 on my TV. A few things I learned along the way:
- I tried the free 'radeon' driver. My monitor worked - TV was displaying color but I couldn't get it synced properly
- the proprietary 'fglrx' driver works but has these problems:
- X is a little unstable. When restarting the X Server (Ctrl-Alt-Backspace) sometimes it won't restart properly.. requiring a reboot >:(
- The Xv video overlay (Which Myth Uses) Stretches the TV Vertically so you only see half. Ati need to fix this in their driver.
- The OpenGL Overlay consumes too much resources and the TV gets framey
- I had to use the textured video to get it working... although I was seeing blue people!
- Make sure you restart x properly. A Logout doesn't work. Use Ctrl-Alt-Backspace or /etc/init.d/gdm restart
A good guide for installing ATI drivers can be found here: Ubuntu_Edgy_Installation_Guide
Installation
- Go to ATI's website and download their driver. chmod 755 and run it as sudo.
- Set up your /etc/X11/xorg.conf
- Restart your x-server
That should have your tv out working at least. Reboot if necessary and have your svideo plugged into your tv and make sure your tv is on so that it can autodetect. You should see the bootsplash on both your monitor and tv. (Funnily enough the TV seems to be updated faster than my monitor!). The following is tweaks made to your xorg.conf file to get myth running smoothly on your tv out:
Here are the interesting parts of my xorg.conf with the extras I added in bold:
Drivers Dvico Input Devices Device
Blue People Fix
I sourced this from the following thread: Overlay on TV-Out stretched
When you use the texturedvideo overlay you will probably see blue people. To get around this problem there is a flag in the myth source to use ATI's drivers: USE_ATI_PROPRIETARY_DRIVER_XVIDEO_HACK. Luckily some nice folks have already compiled it for us. You can get it here:LibMyth0.20.
- Download it to your desktop and run it.
- Stop the backend server, run myth setup then quit, restart the back end server and then try it out:
If all goes well you should see no more smurfs
DVD Ripping
This is very useful when you don't want your kids scratching up their (or your) DVD's. You need to run mtd (Myth Transcoding Demon) before mythfrontend for this to work. However if you have protected DVD's this wont work just yet. For example throw in one of your dvd's and type mtd. You will probably see the warning that libdvdread : can't do encrypted files. To get around this run this script as root:
Run mtd again and everything should be OK. Now to have mtd start up in the background each boot add 'mtd -d' to rc.local.
Drivers Dvico Input Devices
/etc/rc.local
Connecting to Your MythBox Remotely
If your like me and you dont have a monitor attached to your mythbox you can easily connect to your Desktop using VNC.On your desktop go to:
- System > Preferences > Remote Desktop
- Make sure all of these are checked
- [checked] Allow Others to View your desktop
- [checked] Allow Others to Control your desktop
- [checked] Reqire the user to enter this password [******]
Thats it for the MythBox. Now you can connect from your remote computer (Windows / Linux / Java) using this free small footprint tool: Tight VNC
Drivers Dvico Input Devices Input
Recommended Reading
This Wiki
External
Drivers Dvico Input Devices Using
